Written by Isaac Grossberg, Software Engineer
Read Part 2 of our FactoryTalk Optix series about OOP.
One of the best things about using Rockwell Automation’s FactoryTalk Optix platform for developing HMIs is how you can tweak and enhance functionality through NetLogic. This feature lets you tap directly into the .NET framework to supercharge what you can do. And trust me, once you get the hang of it, it’s a game-changer.
What is NetLogic?
NetLogic acts as your backstage pass in Optix, opening the door between the user-friendly graphical interface of the Studio and the software engine powering Optix. You can attach NetLogic nodes to any other node in the system. Think of these nodes as hubs where you can develop methods, giving you access to custom code that makes your HMI behave exactly how you want.
RunTime vs DesignTime
There are two flavors of NetLogic, and which one you use depends on when you want it to execute. RunTime NetLogic scripts make changes that only apply during runtime, without touching the project structure permanently (except for data retained via the Retentivity feature). DesignTime NetLogic, on the other hand, is designed (no pun intended) to get down to business while you’re still in Studio, making lasting changes to the project structure outside the runtime environment.
NetLogic Fundamentals
Ready to add some NetLogic to your project? Just right-click any node in Studio, then select New > [RunTime/DesignTime] NetLogic. Adding new methods is as easy as right-clicking your NetLogic node and choosing New > Method.
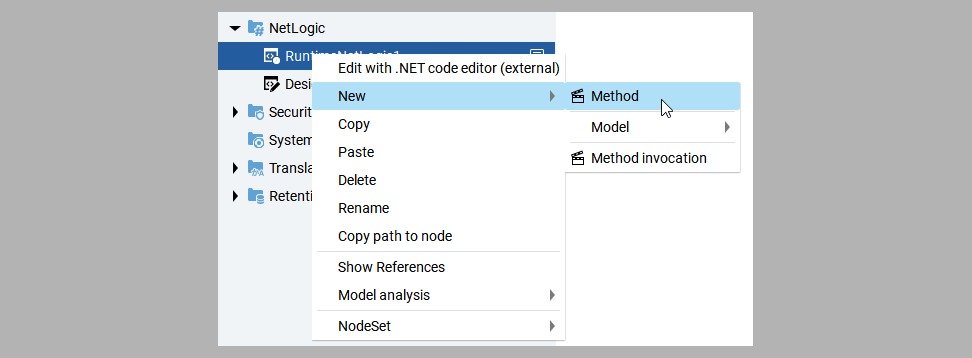
When you double-click a NetLogic object in Studio, you’ll want an IDE (Integrated Development Environment) ready to dig into the code. I highly recommend Visual Studio for this.
By default, two methods are generated for you: Start and Stop. The Start method triggers as soon as the NetLogic’s parent object renders on the screen, while the Stop method kicks in when the parent object leaves.
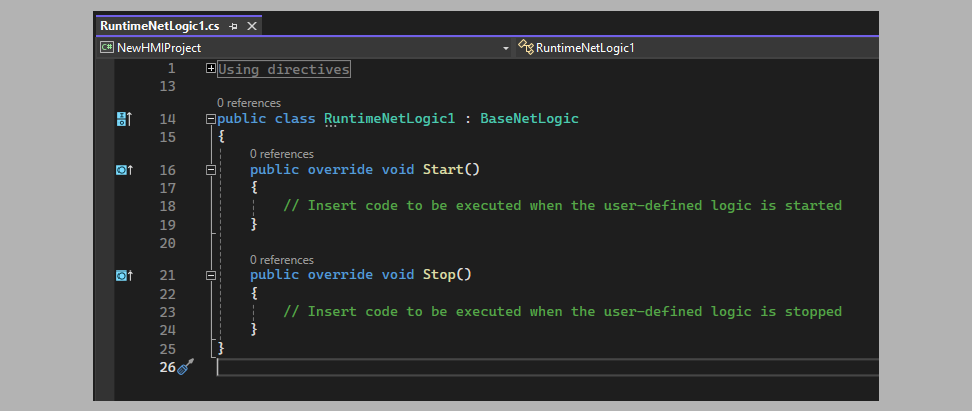
As you add more methods in Studio, each gets tagged with [ExportMethod], which means they’re ready to be used within Studio. If you’re familiar with C# or other object-oriented languages, you will know that you can define additional methods on the NetLogic class for internal calculation. This isn’t necessary but can help write cleaner code.
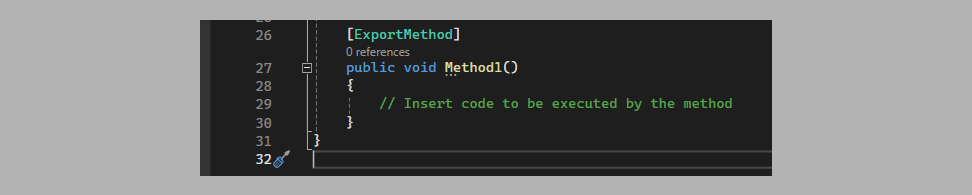
Exported Methods can be dynamically linked to events in Studio, similar to any of the base methods provided by Optix out of the box.
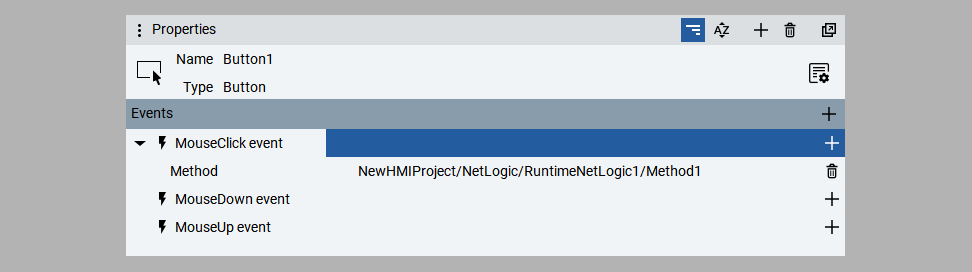
Example Application 1: Array Filtering
Let’s talk about ComboBoxes. Ever tried to display a dynamic list from a PLC string array, but Optix insists on showing the entire array, even the empty values? Annoying, right? This is where NetLogic steps in to save the day.
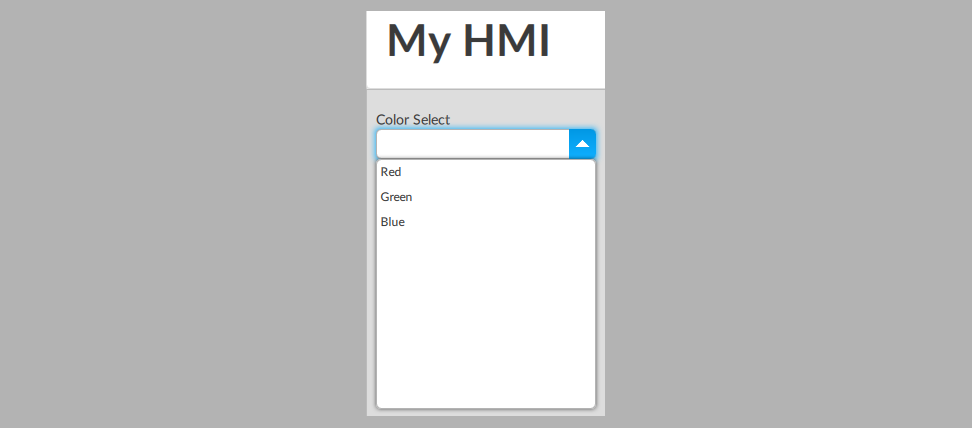
You can write a NetLogic script to filter out those pesky empty or null values before displaying them in the ComboBox. The result? A cleaner, more dynamic dropdown.
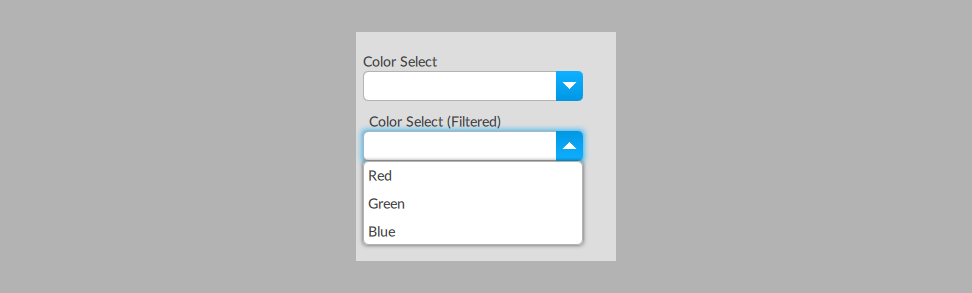
Here’s the code that makes it happen.
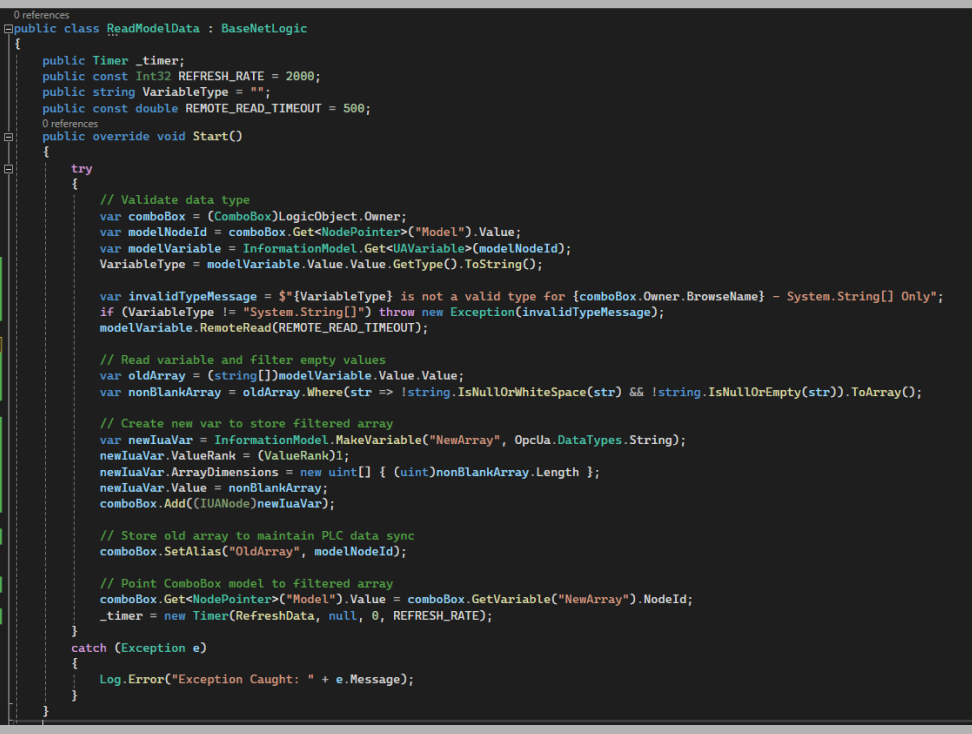
To make things even smoother, you can turn this ComboBox+NetLogic component into a reusable type. Now all you need to do is hook up the array variable to the model property, and the selected string variable to the selected item and value properties.
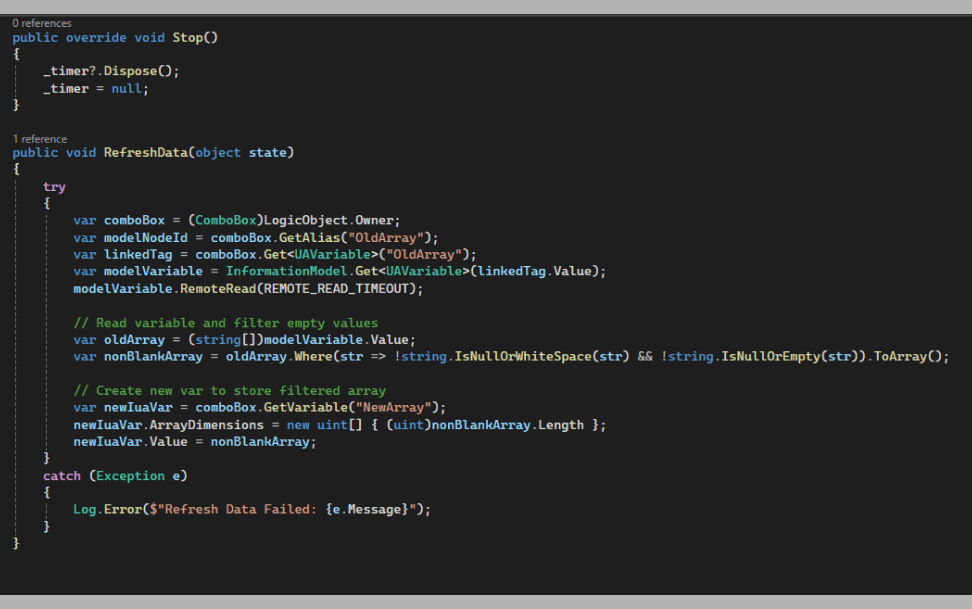
Example Application 2: Delayed Modal Close for Momentary Button
Now let’s take a look at another common challenge: Momentary Buttons and Dialogs. It’s standard to use a Dialog as a confirmation pop-up before an action, and to hold a flag (Boolean) high for a set time—say, 500ms—using a Momentary Button. But here’s the tricky part: You want the Dialog’s confirmation button to trigger both the flag and close the Dialog at the same time.
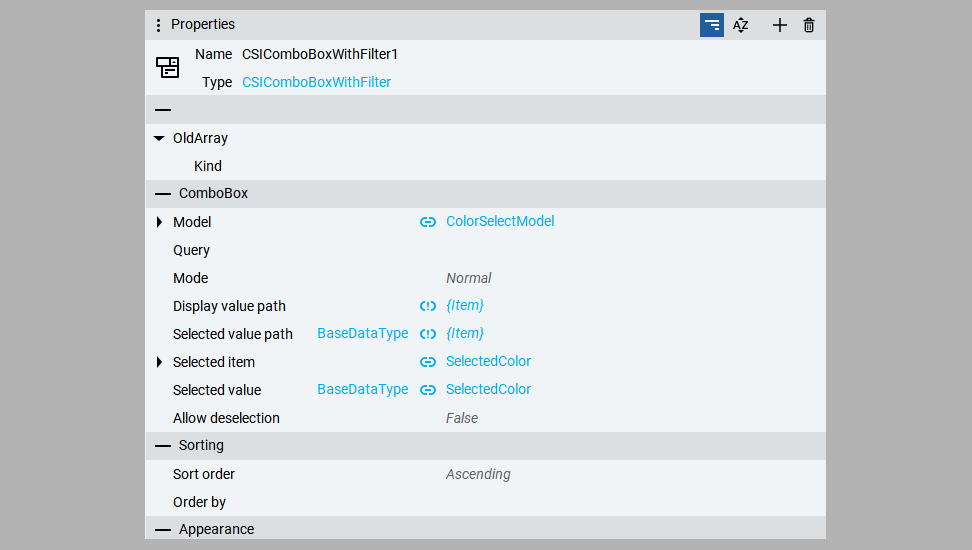
At first, assigning the flag to the active property of the Momentary Button and assigning the Dialog’s Close method to the mouse-click event seems like it works. But you might notice something odd—the flag will get stuck high. That’s because when the Dialog closes, it wipes out the NetLogic context before the script can finish.
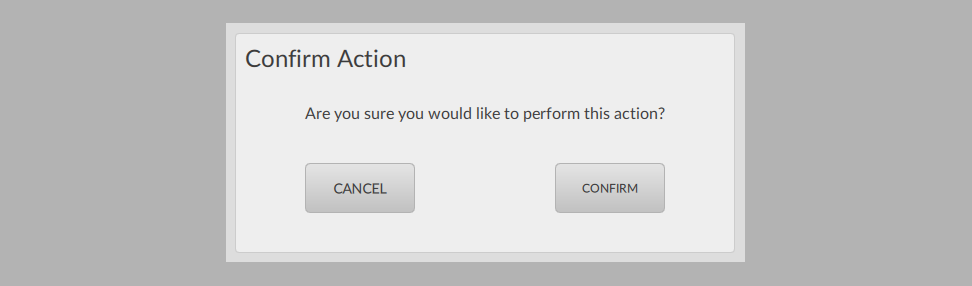
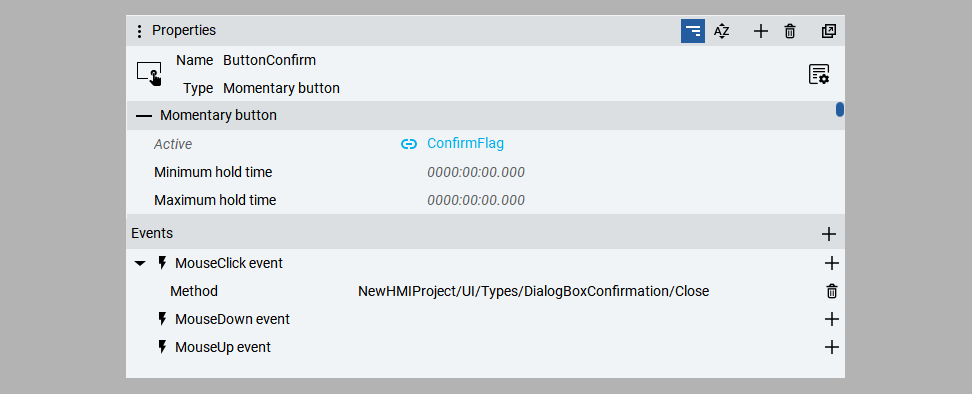
The solution? Write a NetLogic script tied to the Button that holds the flag high for the right amount of time before closing the Dialog. Here’s how to do it.
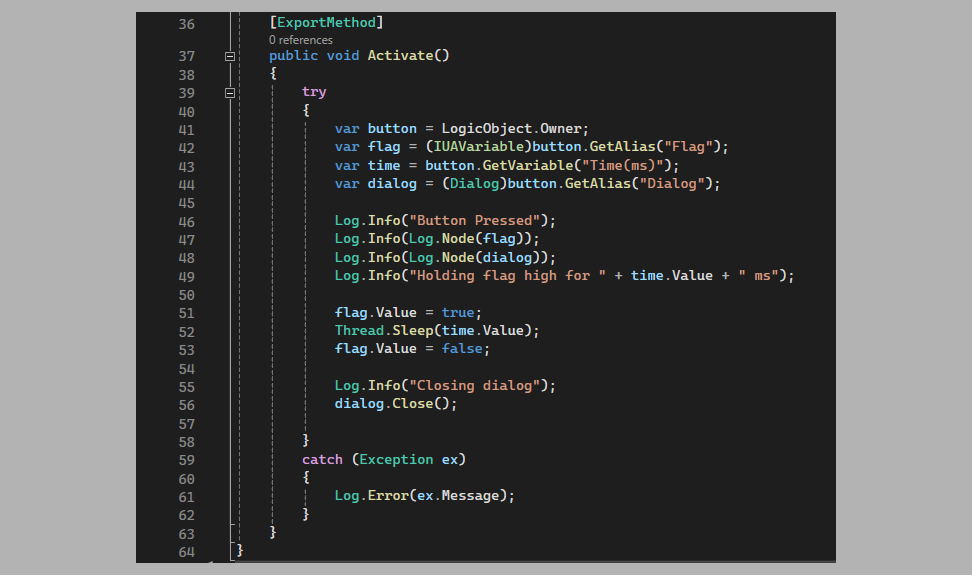
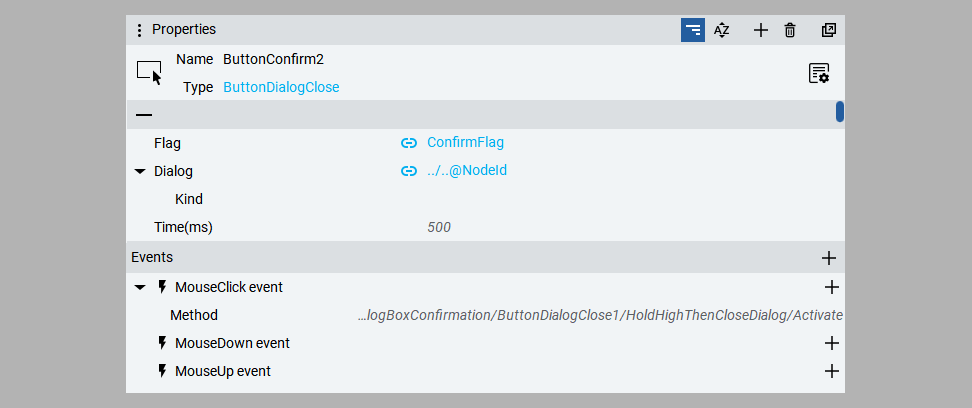
And just like with the ComboBox, you can turn this component into a reusable type for your project.
Example Application 3: Programmatic Generation of UI
Let’s shift gears and talk about DesignTime NetLogic—it’s where things really start to get interesting. You’ve been hands-on with RunTime NetLogic, but now it’s time to level up by programmatically generating your user interface and variable logic. This is where the power of the Optix-type system kicks in, and you can automate some serious magic.
First things first, you need to set up the basics. You’ll need at least one UI type, one model type, and one container to get started. Remember the car monitor example from FactoryTalk Optix Part 2? That’s the kind of setup you’ll want to mimic here.
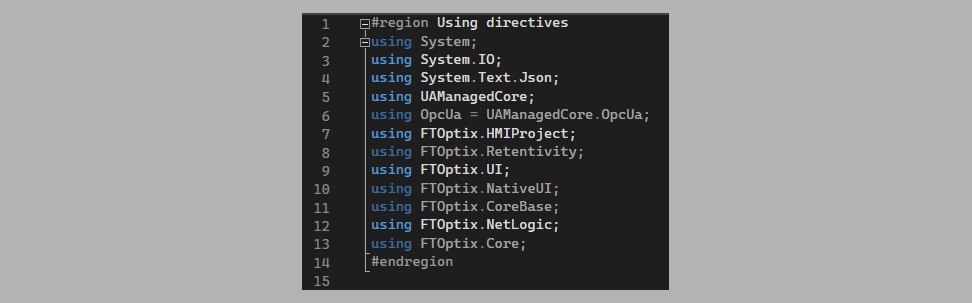
Next, you’ll need a specification file for your automated generation system. Format isn’t a big deal—you can use JSON for this example. To navigate your file system and read that JSON file, just include a couple of directives at the top of your NetLogic file.

Once your JSON structure is set, define it as a new class in your file. You’ll assign get and set methods for each data point. Then, simply read the file into an instance of that class. After that, grab your model type, model destination node, UI type, and UI destination node by right-clicking them in Studio and selecting Copy Path to Node. Trim off the project name, store those paths as static constants, and you’re ready to roll. Use the number defined in the file to loop through the process of generating new instances of your model and UI components, linking them together, and dropping them into the right destinations.
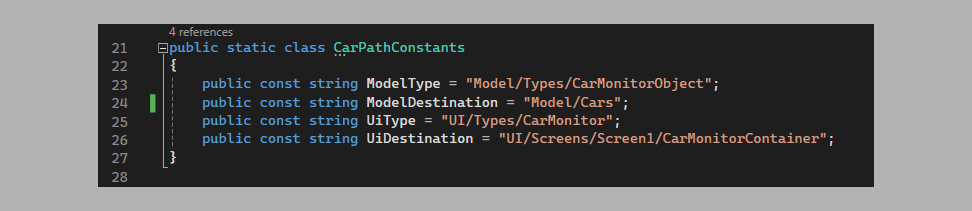
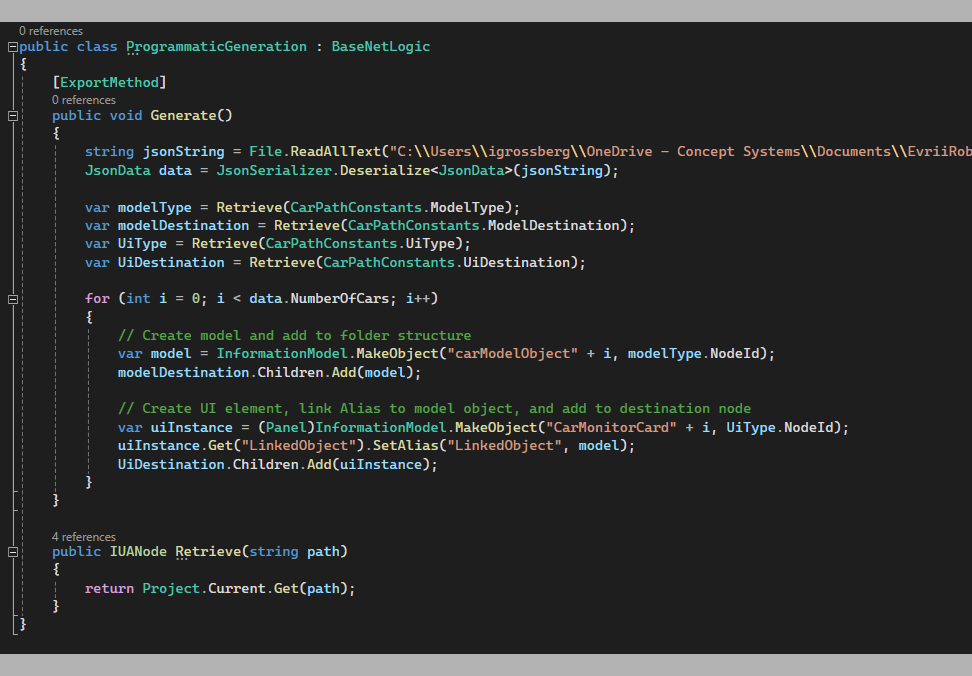
Finally, open up your project and make sure all your components and model variables are in the right spots. Pro tip: Place your UI components in responsive container types like HorizontalLayout or VerticalLayout. This way, you can handle a dynamic number of children without breaking your application. Make sure wrapping is turned on for your layout containers so they don’t overflow the screen!
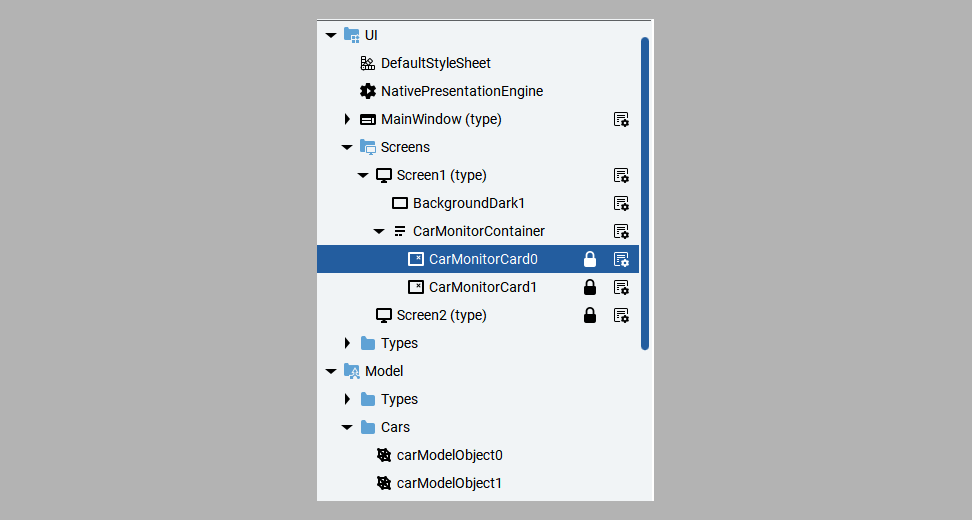
Conclusion
NetLogic is hands-down one of the most powerful tools in FactoryTalk Optix. It allows you to customize and overcome nearly any software limitation. Now that you’ve got a feel for it, you’re ready to push your projects further. Stay tuned for the next and final post, where we’ll dive into Dynamic Links in FactoryTalk Optix.